Mạng xã hội đang phát triển nhảy vọt, đó là lý do vì sao ngày càng có nhiều trang web đang cố gắng tận dụng lợi thế này bằng cách tích hợp vào website. Trong bài viết này, iTOOL sẽ hướng dẫn bạn tạo một ứng dụng đơn giản đăng nhập bằng Facebook tích hợp vào website.
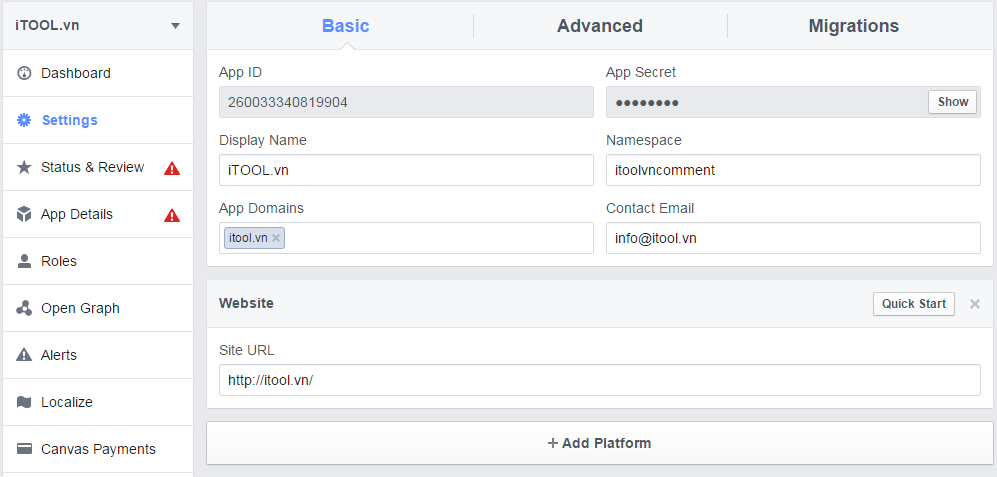
Tiếp theo bạo tạo một class như bên dưới để có thể lấy các thông tin người dùng được Facebook cung cấp.
Khi người sử dụng xác thực đối với Facebook, facebook sẽ gửi truy cập Token, mà chúng ta có thể sử dụng thay mặt cho người sử dụng để thực hiện các yêu cầu hơn nữa để Facebook.
Bạn có thể xem Demo - signin với Facebook trong ASP.NET.
Tạo Facebook Application ID
Đầu tiên bạn truy cập vào https://developers.facebook.com tạo một ứng dụng vào điền các thông tin như hình bên dưới. Nếu bạn test trên Local thì App Domains sửa lại thành localhost.public class FacebookUser
{
public long id
{ get; set; }
public string email
{ get; set; }
public string name
{ get; set; }
public string first_name
{ get; set; }
public string last_name
{ get; set; }
public string gender
{ get; set; }
public string link
{ get; set; }
public DateTime updated_time
{ get; set; }
public DateTime birthday
{ get; set; }
public string locale
{ get; set; }
public string picture
{ get; set; }
public FacebookLocation location
{ get; set; }
}
public class FacebookLocation
{
public string id
{ get; set; }
public string name
{ get; set; }
}
{
public long id
{ get; set; }
public string email
{ get; set; }
public string name
{ get; set; }
public string first_name
{ get; set; }
public string last_name
{ get; set; }
public string gender
{ get; set; }
public string link
{ get; set; }
public DateTime updated_time
{ get; set; }
public DateTime birthday
{ get; set; }
public string locale
{ get; set; }
public string picture
{ get; set; }
public FacebookLocation location
{ get; set; }
}
public class FacebookLocation
{
public string id
{ get; set; }
public string name
{ get; set; }
}
Khi người sử dụng xác thực đối với Facebook, facebook sẽ gửi truy cập Token, mà chúng ta có thể sử dụng thay mặt cho người sử dụng để thực hiện các yêu cầu hơn nữa để Facebook.
Mã cho trang default.aspx:
<body>
<div id="fb-root"></div>
<a href="#" onclick="loginByFacebook();">Login with Facebook</a>
<script type="text/javascript">
window.fbAsyncInit = function () {
FB.init({ appId: 'App Id',
status: true, // check login status
cookie: true, // enable cookies to allow the server to access the session
xfbml: true, // parse XFBML
oauth: true // enable OAuth 2.0
});
};
(function () {
var e = document.createElement('script'); e.async = true;
e.src = document.location.protocol +
'//connect.facebook.net/en_US/all.js';
document.getElementById('fb-root').appendChild(e);
} ());
function loginByFacebook() {
FB.login(function (response) {
if (response.authResponse) {
FacebookLoggedIn(response);
} else {
console.log('User cancelled login or did not fully authorize.');
}
}, { scope: 'user_birthday,user_about_me,user_hometown,user_location,email,publish_stream' });
}
function FacebookLoggedIn(response) {
var loc = '/callback.aspx';
if (loc.indexOf('?') > -1)
window.location = loc + '&authprv=facebook&access_token=' + response.authResponse.accessToken;
else
window.location = loc + '?authprv=facebook&access_token=' + response.authResponse.accessToken;
}
</script>
</body>
<div id="fb-root"></div>
<a href="#" onclick="loginByFacebook();">Login with Facebook</a>
<script type="text/javascript">
window.fbAsyncInit = function () {
FB.init({ appId: 'App Id',
status: true, // check login status
cookie: true, // enable cookies to allow the server to access the session
xfbml: true, // parse XFBML
oauth: true // enable OAuth 2.0
});
};
(function () {
var e = document.createElement('script'); e.async = true;
e.src = document.location.protocol +
'//connect.facebook.net/en_US/all.js';
document.getElementById('fb-root').appendChild(e);
} ());
function loginByFacebook() {
FB.login(function (response) {
if (response.authResponse) {
FacebookLoggedIn(response);
} else {
console.log('User cancelled login or did not fully authorize.');
}
}, { scope: 'user_birthday,user_about_me,user_hometown,user_location,email,publish_stream' });
}
function FacebookLoggedIn(response) {
var loc = '/callback.aspx';
if (loc.indexOf('?') > -1)
window.location = loc + '&authprv=facebook&access_token=' + response.authResponse.accessToken;
else
window.location = loc + '?authprv=facebook&access_token=' + response.authResponse.accessToken;
}
</script>
</body>
Mã cho callback.aspx
Ở đây chúng ta đang lấy thêm thông tin chi tiết của người dùng để hiển thị nó (hoặc bạn có thể lưu nó trong cơ sở dữ liệu của bạn).public partial class callback : System.Web.UI.Page
{
//TODO: paste your facebook-app key here!!
public const string FaceBookAppKey = "XXXXXXXXXXXXX";
protected void Page_Load(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(Request.QueryString["access_token"])) return; //ERROR! No token returned from Facebook!!
//let's send an http-request to facebook using the token
string json = GetFacebookUserJSON(Request.QueryString["access_token"]);
//and Deserialize the JSON response
JavaScriptSerializer js = new JavaScriptSerializer();
FacebookUser oUser = js.Deserialize<FacebookUser>(json);
if (oUser != null)
{
Response.Write("Welcome, " + oUser.name);
Response.Write("<br />id, " + oUser.id);
Response.Write("<br />email, " + oUser.email);
Response.Write("<br />first_name, " + oUser.first_name);
Response.Write("<br />last_name, " + oUser.last_name);
Response.Write("<br />gender, " + oUser.gender);
Response.Write("<br />link, " + oUser.link);
Response.Write("<br />updated_time, " + oUser.updated_time);
Response.Write("<br />birthday, " + oUser.birthday);
Response.Write("<br />locale, " + oUser.locale);
Response.Write("<br />picture, " + oUser.picture);
if (oUser.location != null)
{
Response.Write("<br />locationid, " + oUser.location.id);
Response.Write("<br />location_name, " + oUser.location.name);
}
}
}
/// <summary>
/// sends http-request to Facebook and returns the response string
/// </summary>
private static string GetFacebookUserJSON(string access_token)
{
string url = string.Format("https://graph.facebook.com/me?access_token={0}&fields=email,name,first_name,last_name,link", access_token);
WebClient wc = new WebClient();
Stream data = wc.OpenRead(url);
StreamReader reader = new StreamReader(data);
string s = reader.ReadToEnd();
data.Close();
reader.Close();
return s;
}
}
{
//TODO: paste your facebook-app key here!!
public const string FaceBookAppKey = "XXXXXXXXXXXXX";
protected void Page_Load(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(Request.QueryString["access_token"])) return; //ERROR! No token returned from Facebook!!
//let's send an http-request to facebook using the token
string json = GetFacebookUserJSON(Request.QueryString["access_token"]);
//and Deserialize the JSON response
JavaScriptSerializer js = new JavaScriptSerializer();
FacebookUser oUser = js.Deserialize<FacebookUser>(json);
if (oUser != null)
{
Response.Write("Welcome, " + oUser.name);
Response.Write("<br />id, " + oUser.id);
Response.Write("<br />email, " + oUser.email);
Response.Write("<br />first_name, " + oUser.first_name);
Response.Write("<br />last_name, " + oUser.last_name);
Response.Write("<br />gender, " + oUser.gender);
Response.Write("<br />link, " + oUser.link);
Response.Write("<br />updated_time, " + oUser.updated_time);
Response.Write("<br />birthday, " + oUser.birthday);
Response.Write("<br />locale, " + oUser.locale);
Response.Write("<br />picture, " + oUser.picture);
if (oUser.location != null)
{
Response.Write("<br />locationid, " + oUser.location.id);
Response.Write("<br />location_name, " + oUser.location.name);
}
}
}
/// <summary>
/// sends http-request to Facebook and returns the response string
/// </summary>
private static string GetFacebookUserJSON(string access_token)
{
string url = string.Format("https://graph.facebook.com/me?access_token={0}&fields=email,name,first_name,last_name,link", access_token);
WebClient wc = new WebClient();
Stream data = wc.OpenRead(url);
StreamReader reader = new StreamReader(data);
string s = reader.ReadToEnd();
data.Close();
reader.Close();
return s;
}
}
Bạn có thể xem Demo - signin với Facebook trong ASP.NET.